Exploring SHA and MD5: Essential Cryptographic Hash Functions for Developers
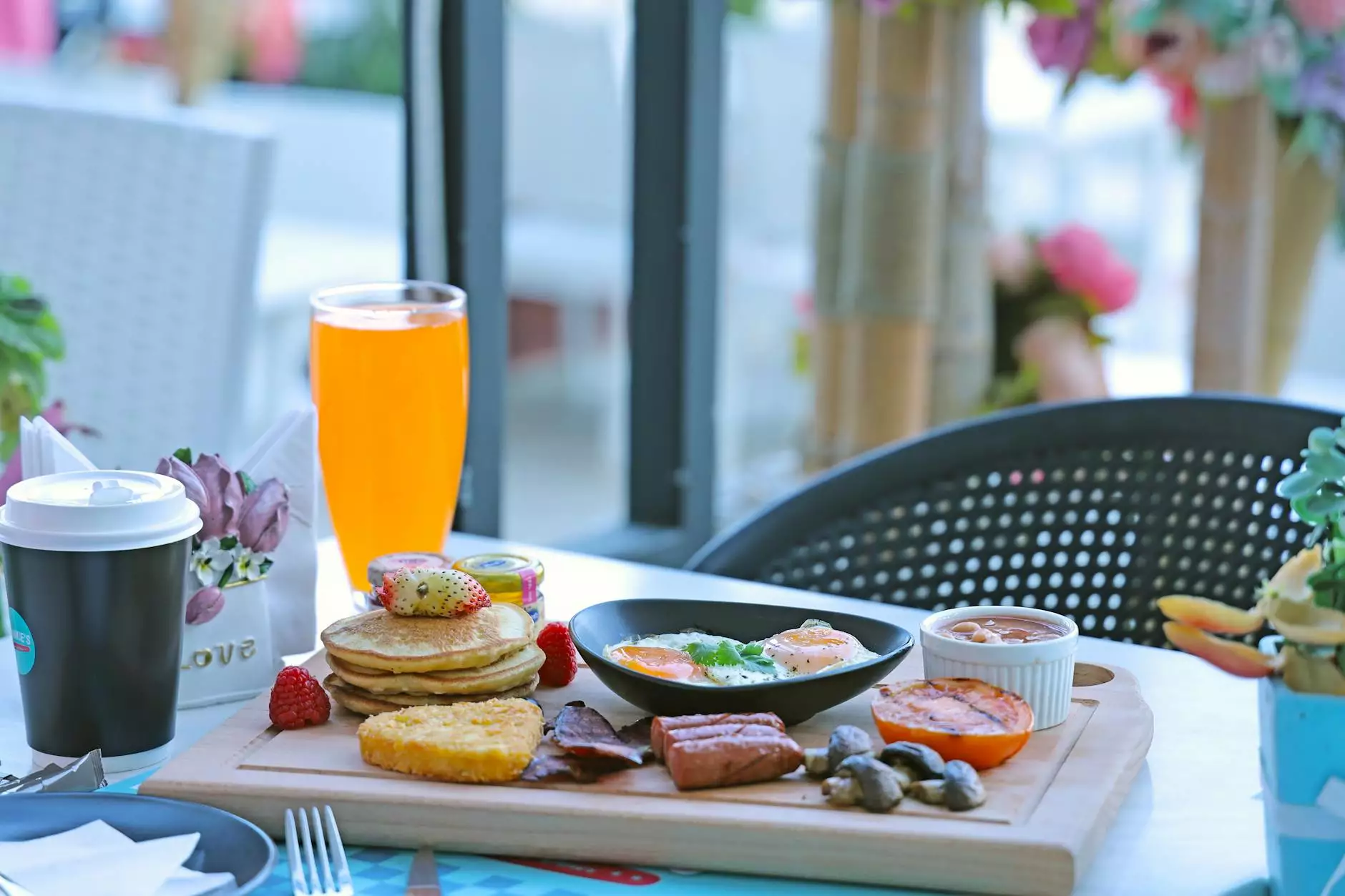
In today's digital landscape, cryptography plays a pivotal role in securing data and maintaining the integrity of information. Among the many cryptographic algorithms, the SHA (Secure Hash Algorithm) and MD5 (Message-Digest Algorithm 5) are two of the most important hash functions employed in various applications, particularly in the fields of web design and software development.
What are SHA and MD5?
The phrases "SHA MD5" refer to specific cryptographic hash functions used to generate a fixed-size hash value from input data of arbitrary length. This process is known as hashing, and it serves multiple purposes, including:
- Data Integrity: Hash functions help in ensuring that the data has not been altered during transmission.
- Password Storage: Securely storing passwords by hashing them before saving to a database.
- Digital Signatures: Used to verify the authenticity and integrity of digital messages or documents.
Understanding SHA
The SHA family of algorithms was developed by the National Security Agency (NSA) and published by the National Institute of Standards and Technology (NIST). These algorithms vary in length, including SHA-1, SHA-256, and SHA-512, which is currently the most secure and widely used. The Secure Hash Algorithm provides a way to create unique identifiers for data.
Understanding MD5
MD5, developed by Ronald Rivest in 1991, creates a 128-bit hash value. Although it was once widely used for integrity checks and password storage, MD5 is now considered broken and is not recommended for secure applications due to vulnerabilities that allow collision attacks. Despite this, understanding MD5 remains important for legacy systems and applications.
How SHA and MD5 Work
Both SHA and MD5 operate through a series of mathematical operations that process the input data and generate a fixed-size output. Here’s a brief look at their mechanics:
The Hashing Process
- Input Data: Any data, such as a file or a message, is taken as input.
- Preprocessing: The data is padded and split into blocks.
- Compression: Each block is processed to create a condensed representation.
- Output: A fixed-size hash value is produced.
Common Applications of SHA and MD5
Using SHA and MD5 in web design and software development is crucial in maintaining security and data integrity. Here are some common applications:
- Password Hashing: Securely storing user passwords by hashing them using SHA or MD5 to prevent unauthorized access.
- Data Verification: Utilizing hashes to verify the integrity of files during downloads or data transfers.
- API Authentication: Implementing SHA or MD5 hash functions for token generation in secure API authentication protocols.
- Digital Signatures: Creating digital signatures that ensure non-repudiation in electronic transactions.
Implementing SHA and MD5 in Programming Languages
Many programming languages provide libraries and facilities to easily use SHA and MD5 hashing algorithms. Below are examples in some popular languages:
1. Python
Python’s hashlib library makes it straightforward to work with these algorithms:
import hashlib # Using SHA-256 sha256_hash = hashlib.sha256(b"example data").hexdigest() print("SHA-256:", sha256_hash) # Using MD5 md5_hash = hashlib.md5(b"example data").hexdigest() print("MD5:", md5_hash)2. Java
Java provides the MessageDigest class for hashing:
import java.security.MessageDigest; public class HashExample { public static void main(String[] args) throws Exception { MessageDigest md = MessageDigest.getInstance("SHA-256"); byte[] digest = md.digest("example data".getBytes()); System.out.println("SHA-256: " + bytesToHex(digest)); } private static String bytesToHex(byte[] bytes) { StringBuilder sb = new StringBuilder(); for (byte b : bytes) { sb.append(String.format("%02x", b)); } return sb.toString(); } }3. C#
In C#, you can utilize the System.Security.Cryptography namespace:
using System; using System.Security.Cryptography; using System.Text; class Program { static void Main() { using (SHA256 sha256 = SHA256.Create()) { byte[] bytes = sha256.ComputeHash(Encoding.UTF8.GetBytes("example data")); Console.WriteLine("SHA-256: " + BitConverter.ToString(bytes).Replace("-", "").ToLowerInvariant()); } } }4. C++
In C++, you can use libraries such as openssl:
#include #include int main() { unsigned char hash[SHA256_DIGEST_LENGTH]; SHA256_CTX sha256; SHA256_Init(&sha256); SHA256_Update(&sha256, "example data", strlen("example data")); SHA256_Final(hash, &sha256); for(int i = 0; i